This article shows how you can use QTP
to send mails to any email address using email service providers such as
GMail, Yahoo Mail etc. Automatically sending mails through QTP can be a
very nice enhancement to your automation framework. For example, after
you run your test scripts, you need to email test run reports
(number of test scripts passed / failed etc) to the stakeholders. You
can automate this mailing process and send custom reports to the
required people.
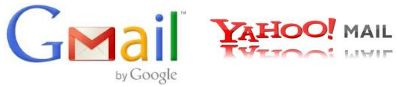
Send Email from Gmail and Yahoo Mail
Before going through the code, lets go through some important points regarding the code -
1. The below given
email code is not directly related to QTP as such. The code is actually a
VBScript function which you can use in QTP. So even if you don’t have
QTP, you can use this VBScript code directly.
2. The email
functionality uses Microsoft’s CDO (Collaboration Data Objects)
technology to send mails. You can find more details about Microsoft CDO
in Microsoft’s MSDN Library.
3. It is not necessary
that you have to use this code using VBScript only. Microsoft CDO can be
programmed using any language that creates COM object such as ASP, VB,
Visual C++, C# etc.
4. The below code has
been tested on Gmail and Yahoo mail. If you want to use this code in
your company’s SMTP server, you need to contact your network team to get
details about the SMTP address, port number etc.
5. To use this code,
you don’t need to have Microsoft Outlook installed on your system. You
can also use MS Outlook to send emails
Let’s now see the code for sending emails using QTP (VBScript) through Gmail.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
| Function fnSendEmail() 'Create an object of CDO type Set myMail=CreateObject( "CDO.Message" ) 'Enable SSL Authentication myMail.Configuration.Fields.Item( "http://schemas.microsoft.com/cdo/configuration/smtpusessl" ) = True 'Enable basic smtp authentication myMail.Configuration.Fields.Item( "http://schemas.microsoft.com/cdo/configuration/smtpauthenticate" ) = 1 'Specify SMTP server and port myMail.Configuration.Fields.Item ( "http://schemas.microsoft.com/cdo/configuration/smtpserver" )= "smtp.gmail.com" myMail.Configuration.Fields.Item ( "http://schemas.microsoft.com/cdo/configuration/smtpserverport" ) = 25 'Specify user id and password myMail.Configuration.Fields.Item( "http://schemas.microsoft.com/cdo/configuration/sendusername" ) = "senders_id@gmail.com" myMail.Configuration.Fields.Item( "http://schemas.microsoft.com/cdo/configuration/sendpassword" ) = "**********" 'Update the configuration fields myMail.Configuration.Fields.Update 'Specify email properties myMail.Subject = "Sending Email from QTP" myMail.From = "senders_id@gmail.com" myMail. To = "some_mail_id_1@gmail.com" myMail.CC = "" myMail.BCC = "" myMail.TextBody = "This is the Text Body" 'Send mail myMail.Send Set myMail = Nothing End Function |
How to send mails from your Yahoo mail id
To send mails using your yahoo mail id, you need to replace the Gmail SMTP address and port number with Yahoo’s SMTP address and port number.
1
2
3
| 'Specify Yahoo SMTP server and Port Number myMail.Configuration.Fields.Item ( "http://schemas.microsoft.com/cdo/configuration/smtpserver" )= "smtp.mail.yahoo.com" myMail.Configuration.Fields.Item ( "http://schemas.microsoft.com/cdo/configuration/smtpserverport" ) = 465 |
How to send mails to multiple people in one go.
To send mails to multiple email ids in one go, you need to just enter multiple mail ids separated by a semi colon(;). Example:
1
2
| myMail. To = "some_mail_id_1@gmail.com; some_other_id@yahoo.com" myMail.CC = "some_mail_id@someaddress.com" |
How to send html content in the mail.
Normally you would not send plain text in emails. You would usually be sending some html content in mails. To do so, you need to use HTMLBody in place of TextBody and include html tags in your text body.
1
2
| 'myMail.TextBody = "This is the Text Body" -> TextBody not to be used myMail.HTMLBody = "<html><table border=1><tr>Row1</tr><tr>Row2</tr></table></html>" |
How to send attachments using the email code.
To add an attachment in you mail, you need to include myMail.AddAttachment line in your code and specify the correct path of the file to be attached. To add more than one attachment, repeat myMail.AddAttachment code required number of times.
1
2
3
4
5
6
7
8
9
10
11
| 'Specify email mail properties myMail.Subject = "Sending Attachment in Mail from QTP" myMail.From = "some_id@gmail.com" myMail. To = "some_other_id@gmail.com" myMail.TextBody= "Mail Content" 'Send 2 attachments in the mail myMail.AddAttachment "D:\Attachment1.txt" myMail.AddAttachment "D:\Attachment2.txt" myMail.Send set myMail=nothing |
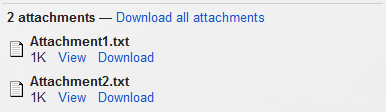
No comments:
Post a Comment